module manager
Important
When there was no built-in KernelSu to obtain root permissions earlier, this function was developed to facilitate the injection of dex and so in a non-root environment. Now that there is a root environment, this function is not so important. You can choose to add the lsposed module to KernelSu.
This function is mainly used to quickly inject dex and so during the startup process. In order to facilitate art hook, the dynamic library of pine is automatically injected internally. Inline hook can be used by configuring dobby injection when developing the module. Here is a simple module example
First create a new android project and modify the AndroidManifest.xml file
<meta-data
android:name="kdesc"
android:value="模块样例" />
<meta-data
android:name="kclass"
android:value="com.example.mysodemo.myModule" />
<meta-data
android:name="ksoname"
android:value="libdobby.so;libmysodemo.so" />
kdesc indicates the description of this field in the module column
kclass represents the entry class started after injecting the module. This class must implement the onStart function in the interface java.lang.krom.IHook
. This function will be called after the module is successfully injected.
ksoname represents the list of dynamic libraries to be injected. In this example, the dynamic libraries of dobby and the current example are automatically injected.
Then add a reference to the pine library in build.gradle
implementation 'top.canyie.pine:core:0.2.6'
After adding reference support, you can create the interface. Create the package path java.lang.krom
in the java directory, and then create the interface file IHook.java
in it.
package java.lang.krom;
public interface IHook {
void onStart(Object app);
}
The interface is ready, just implement it. The following is the implementation of the com.example.mysodemo.myModule
class
public class myModule implements IHook {
public static String TAG="myModule";
@Override
public void onStart(Object app) {
Log.i(TAG,"onStart my demo");
PineConfig.debug = true;
PineConfig.debuggable = BuildConfig.DEBUG;
Log.i(TAG,"start Hook json");
try {
Pine.hook(JSONObject.class.getDeclaredMethod("toString"), new MethodHook() {
@Override public void beforeCall(Pine.CallFrame callFrame) {
Log.i(TAG, "enter json toString");
}
@Override public void afterCall(Pine.CallFrame callFrame) {
Log.i(TAG, "leave json toString "+callFrame.getResult());
}
});
} catch (NoSuchMethodException e) {
throw new RuntimeException(e);
}
}
}
Finally, install the module and enter the module list page again to see this module.
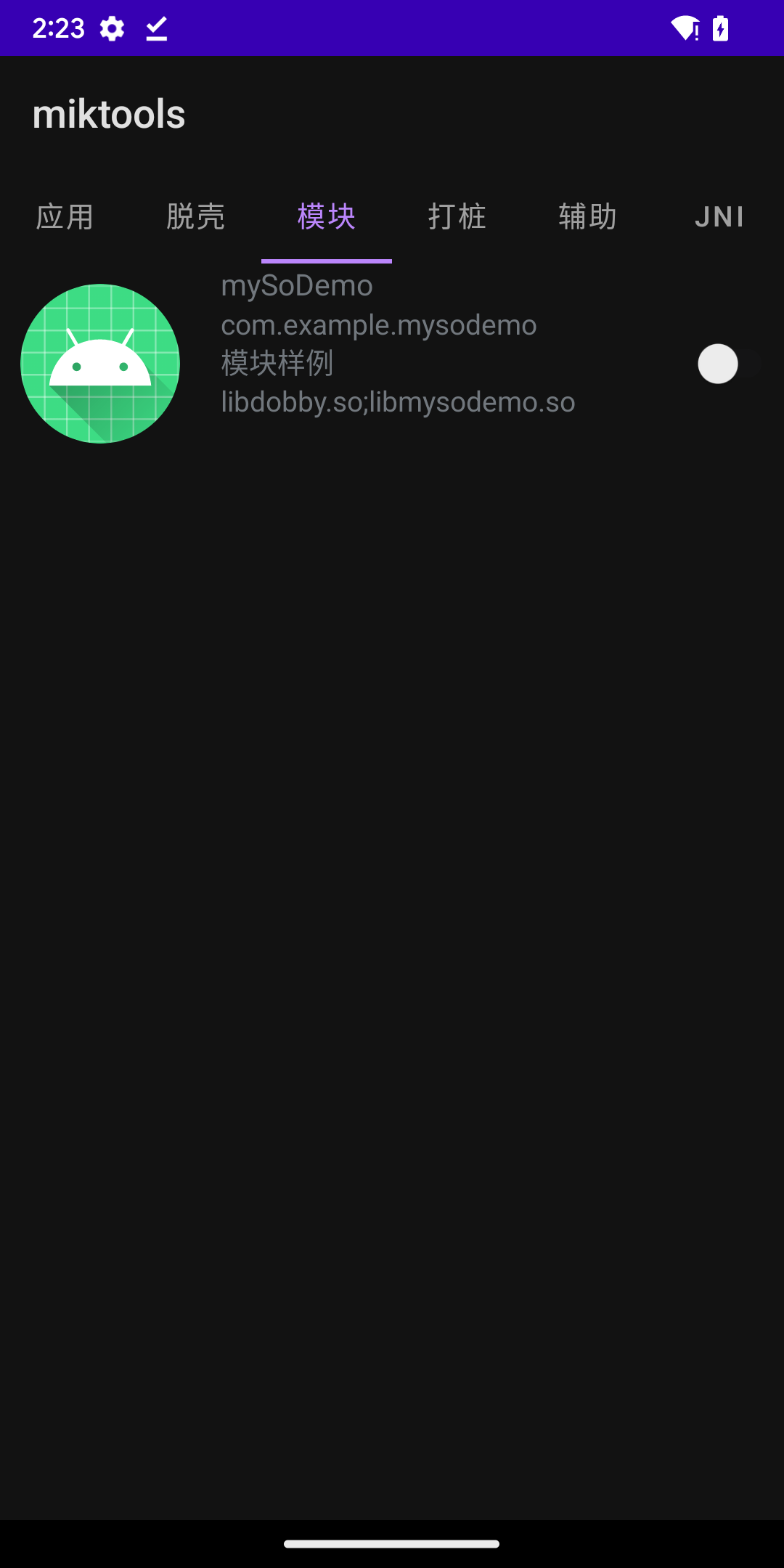
Check this module, resave the configuration, and open the application again to see the following log.
myModule com.aisino.sqzlht I onStart my demo
Since the current system version is based on android13, after the module is modified, when reinstalling, you need to use the command adb install -r app-debug.apk
to update and overwrite this module.
In addition to the IHook
interface, another interface IDeepDex
can also be implemented to complete the docking of in-depth call chain unpacking. The interface is defined as follows
package java.lang.krom;
public interface IDeepDex {
boolean CheckCode(Object[] objs);
}
Let’s look at an example of implementation as follows:
public class testModule implements IHook, IDeepDex {
public static String TAG="testModule";
@Override
public void onStart(Object app) {
Log.i(TAG,"enter onStart");
}
@Override
public boolean CheckCode(Object[] objs) {
String codeItem=(String)objs[0];
int methodIdx=(int)objs[1];
int offset=(int)objs[2];
int codeItemLen=(int)objs[3];
int dexSize=(int)objs[4];
String methodName=objs[5].toString();
if(objs.length>6){
String clsDesc=objs[6].toString();
}
System.out.println("myModule checCode "+codeItem);
return false;
}
}
When opening the in-depth call chain, if a module implements the IDeepDex
interface, its CheckCode
function will be called to let the module determine whether it should be executed deeper. If there is no such module, the default is false, and its execution bytecode will be saved when invoked.
Finally, the module sample address is attached.